API Mocking with WireMock and JSON Server [Basic]
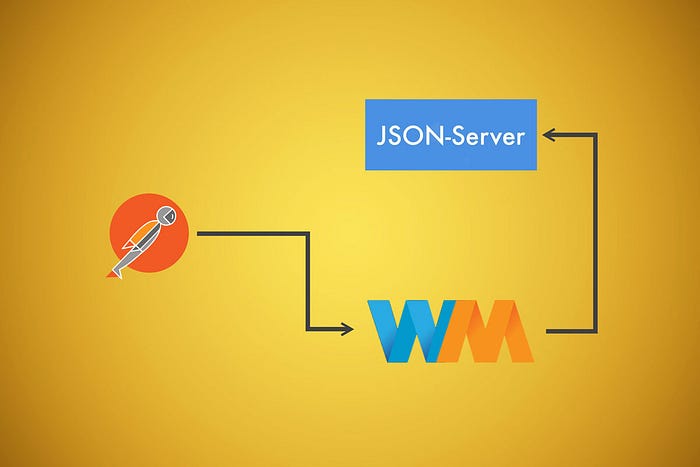
There may be an overwhelmingly complex time for development with every new project or feature, especially if you are developing this new thing with a few different roles or teams. On one side, there is client development like web apps. On the other side, there are services like web APIs.
In the beginning, you are trying to agree on a data contract, but this is one of the well-known promises that can not be held. In such a case, it is tough to sync on changes. It will be a more agile development process if every team works on their own data and gives each other information about changes to synchronize data on unsynchronized timing. Or, on a much more basic scenario, you may want to test/develop with real data or testing servers.
I want to tell you about two great tools and how to combine them with a basic configuration. The tools are suitable for more complex scenarios, but I’ll create a simple scenario in this post. I used WireMock and JSON Server. I show you how to create a basic mock server and edit it to meet your needs.
WireMock
WireMock is an HTTP-based API mocking tool with a great set of features. You can both integrate it with your Java code or use it as a standalone tool. I’ll be using a standalone version. I created a GitHub repo for that and published it in the Docker Hub.
JSON Server
Sometimes we need a REST API with reading and writing features, and I don’t want it to simulate writing. I need to read what I write later. JSON Server is a lifesaver about that. You can provide a JSON file, and it will serve you as a real API, and you can also update this file with REST requests.
Architecture & Process
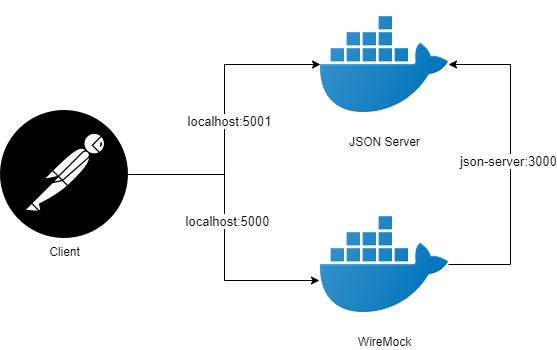
I created a simple architecture that relies on a single docker image for each tool. In this architecture, I expose JSON Server on port 5001, but this is just for testing because we will use WireMock to access the JSON server and mock full service on a single REST API.
You can find all codes and configurations in my API Mocking repo in GitHub.
JSON Server Setup
It is actually straightforward to start with the JSON server. You just need a JSON file as a database to start. I used the following JSON that has posts, comments, and profile data.
It is simple, and if you need more detailed information, here is the GitHub repo. I created a simple docker image for JSON Server and used it in the docker compose
file.
version: "3.9"
services:
json-server:
image: irensaltali/json-server
volumes:
- ./json-data:/json-server
ports:
- "5001:3000"
networks:
- toeachother
This past starts the JSON Server and map json-data
folder to docker so we can use db.json
file in server.
WireMock Setup
I used a standalone version of WireMock and created a docker image from it. WireMock has great detailed features, but I’m going to use only two of them: static response and proxy mapping. I’ll configure it to map JSON Server.
{
"mappings": [
{
"request": {
"method": "GET",
"url": "/test"
},
"response": {
"status": 200,
"body": "More content\n"
}
},
{
"request": {
"method": "ANY",
"urlPattern": "/posts.*"
},
"response": {
"proxyBaseUrl": "http://json-server:3000/"
}
}
]
}
As you see in the JSON file, there are two mappings: first static and the second for proxy. I added the required configuration to docker-compose.yml
so it will run WireMock and use map.json
file.
mock-server:
image: irensaltali/wiremock
volumes:
- ./mappings:/wiremock/mappings
ports:
- "5000:5000"
links:
- "jsonserver:json-server"
depends_on:
- json-server
networks:
- toeachother
Network
In the planned architecture, I need a connection between docker images, so I create a link for that and add this in docker-compose.yml
file.
links:
- "jsonserver:json-server"
Full version of docker-compose.yml
:
Run Servers
You can clone the GitHub repo and run it. If you need any modification on the data of the JSON Server, you can edit json-data/db.json
and for the WireMock mappings, you can edit mappings/map.json.
git clone https://github.com/irensaltali/api-mocking.git
docker network create toeachother
docker compose up
Test
I sent requests with Postman like below:
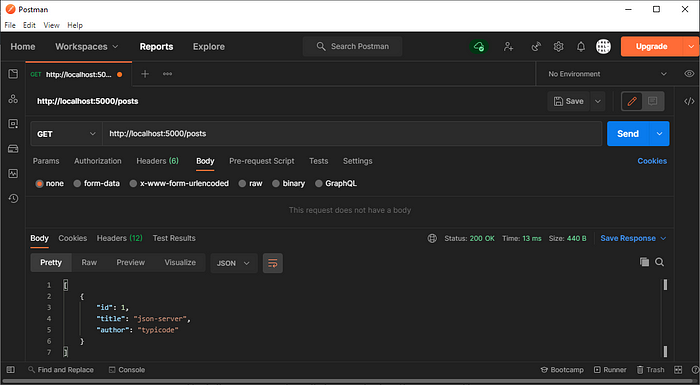
It is a simple GET request to WireMock. WireMock just proxy it to JSON Server, and we get what we wrote in db.json
. We can also update the post by sending a POST request.

We updated the JSON database, and we got a new Id as a response. Now we can request what we wrote with a GET request. Here is the result of a new GET request
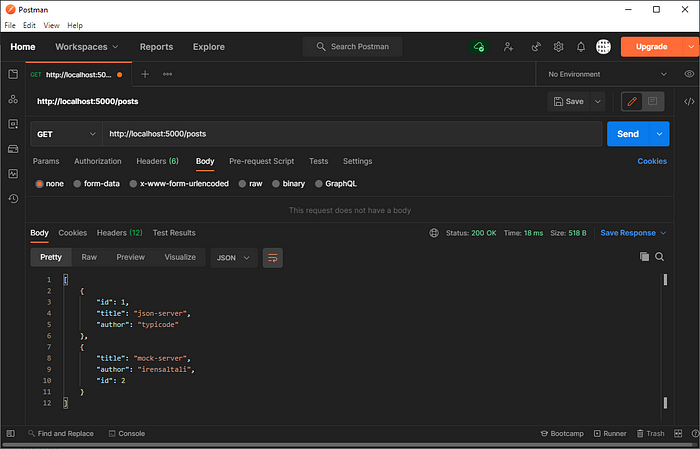
Conclusion
Mocking API calls is a critical practice while developing applications, and as we could see, it’s easy to create mocks on WireMock and JSON Server. This technique introduces several advantages, including, but not limited to, faster development and saving of computing resources.
WireMock and JSON Server features are not limited to what I show you here. Please see their documentation for further information.
Before you go…
If you found this article helpful, click the💚 or 👏 button below or share the article on Twitter so your friends can benefit from it too.
All Codes, Configurations, and Images
- API Mocking repository
- WireMock
- JSON-Server
- WireMock Image in Docker Hub
- JSON-Server Image in Docker Hub
Member discussion